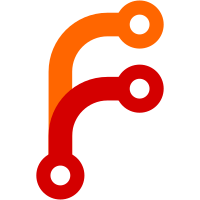
* fix: fix improper authorization when accessing with third-party application
* refactor: refactor type definitions
* fix: get rid of unnecessary access limitation
* enhance: サードパーティアプリケーションがWebsocket APIを使えるように
* fix: add missing parentheses
* Revert "fix(backend): add missing kind definition for admin endpoints to improve security"
This reverts commit 5150053275
.
* frontend: 翻訳の抜けを訂正, read:adminとwrite:adminはアクセス発行トークンのデフォルトでは非表示にする
* enhance(test): misskey-ghsa-7pxq-6xx9-xpgmに関するテストを追加
* enhance(test): Websocket APIに対するテストも追加
* enhance(refactor): `@/misc/api-permissions.ts`を`misskey-js/permissions`に統合
* fix(frontend): アクセストークン発行UIで全ての権限を有効にした際、管理者用APIへのアクセスも許可してしまう問題を修正
* enhance(backend): Websocketの接続に最低限必要な権限を変更
* fix(backend): `/api/admin/meta`をサードパーティアプリケーションからはアクセスできないように
* fix(backend): エンドポイントにアクセスするために必要な権限を変更
* fix(frontend/locale): Add missing type declaration
* chore: update `misskey-js/src/autogen`
---------
Co-authored-by: tamaina <tamaina@hotmail.co.jp>
86 lines
2 KiB
TypeScript
86 lines
2 KiB
TypeScript
/*
|
|
* SPDX-FileCopyrightText: syuilo and other misskey contributors
|
|
* SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
import { bindThis } from '@/decorators.js';
|
|
import type Connection from './Connection.js';
|
|
|
|
/**
|
|
* Stream channel
|
|
*/
|
|
// eslint-disable-next-line import/no-default-export
|
|
export default abstract class Channel {
|
|
protected connection: Connection;
|
|
public id: string;
|
|
public abstract readonly chName: string;
|
|
public static readonly shouldShare: boolean;
|
|
public static readonly requireCredential: boolean;
|
|
public static readonly kind?: string | null;
|
|
|
|
protected get user() {
|
|
return this.connection.user;
|
|
}
|
|
|
|
protected get userProfile() {
|
|
return this.connection.userProfile;
|
|
}
|
|
|
|
protected get following() {
|
|
return this.connection.following;
|
|
}
|
|
|
|
protected get userIdsWhoMeMuting() {
|
|
return this.connection.userIdsWhoMeMuting;
|
|
}
|
|
|
|
protected get userIdsWhoMeMutingRenotes() {
|
|
return this.connection.userIdsWhoMeMutingRenotes;
|
|
}
|
|
|
|
protected get userIdsWhoBlockingMe() {
|
|
return this.connection.userIdsWhoBlockingMe;
|
|
}
|
|
|
|
protected get userMutedInstances() {
|
|
return this.connection.userMutedInstances;
|
|
}
|
|
|
|
protected get followingChannels() {
|
|
return this.connection.followingChannels;
|
|
}
|
|
|
|
protected get subscriber() {
|
|
return this.connection.subscriber;
|
|
}
|
|
|
|
constructor(id: string, connection: Connection) {
|
|
this.id = id;
|
|
this.connection = connection;
|
|
}
|
|
|
|
@bindThis
|
|
public send(typeOrPayload: any, payload?: any) {
|
|
const type = payload === undefined ? typeOrPayload.type : typeOrPayload;
|
|
const body = payload === undefined ? typeOrPayload.body : payload;
|
|
|
|
this.connection.sendMessageToWs('channel', {
|
|
id: this.id,
|
|
type: type,
|
|
body: body,
|
|
});
|
|
}
|
|
|
|
public abstract init(params: any): void;
|
|
|
|
public dispose?(): void;
|
|
|
|
public onMessage?(type: string, body: any): void;
|
|
}
|
|
|
|
export type MiChannelService<T extends boolean> = {
|
|
shouldShare: boolean;
|
|
requireCredential: T;
|
|
kind: T extends true ? string : string | null | undefined;
|
|
create: (id: string, connection: Connection) => Channel;
|
|
}
|