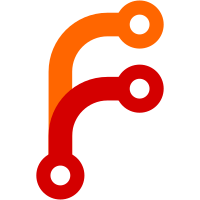
* fix: hide masked value The ::add-mask:: command output logs the value to be masked. This does expose critical information which should be hidden from the output. * Add test to not output secret in add-mask command Co-authored-by: mergify[bot] <37929162+mergify[bot]@users.noreply.github.com>
106 lines
2.7 KiB
Go
106 lines
2.7 KiB
Go
package runner
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/nektos/act/pkg/common"
|
|
"github.com/sirupsen/logrus/hooks/test"
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestSetEnv(t *testing.T) {
|
|
a := assert.New(t)
|
|
ctx := context.Background()
|
|
rc := new(RunContext)
|
|
handler := rc.commandHandler(ctx)
|
|
|
|
handler("::set-env name=x::valz\n")
|
|
a.Equal("valz", rc.Env["x"])
|
|
}
|
|
|
|
func TestSetOutput(t *testing.T) {
|
|
a := assert.New(t)
|
|
ctx := context.Background()
|
|
rc := new(RunContext)
|
|
rc.StepResults = make(map[string]*stepResult)
|
|
handler := rc.commandHandler(ctx)
|
|
|
|
rc.CurrentStep = "my-step"
|
|
rc.StepResults[rc.CurrentStep] = &stepResult{
|
|
Outputs: make(map[string]string),
|
|
}
|
|
handler("::set-output name=x::valz\n")
|
|
a.Equal("valz", rc.StepResults["my-step"].Outputs["x"])
|
|
|
|
handler("::set-output name=x::percent2%25\n")
|
|
a.Equal("percent2%", rc.StepResults["my-step"].Outputs["x"])
|
|
|
|
handler("::set-output name=x::percent2%25%0Atest\n")
|
|
a.Equal("percent2%\ntest", rc.StepResults["my-step"].Outputs["x"])
|
|
|
|
handler("::set-output name=x::percent2%25%0Atest another3%25test\n")
|
|
a.Equal("percent2%\ntest another3%test", rc.StepResults["my-step"].Outputs["x"])
|
|
|
|
handler("::set-output name=x%3A::percent2%25%0Atest\n")
|
|
a.Equal("percent2%\ntest", rc.StepResults["my-step"].Outputs["x:"])
|
|
|
|
handler("::set-output name=x%3A%2C%0A%25%0D%3A::percent2%25%0Atest\n")
|
|
a.Equal("percent2%\ntest", rc.StepResults["my-step"].Outputs["x:,\n%\r:"])
|
|
}
|
|
|
|
func TestAddpath(t *testing.T) {
|
|
a := assert.New(t)
|
|
ctx := context.Background()
|
|
rc := new(RunContext)
|
|
handler := rc.commandHandler(ctx)
|
|
|
|
handler("::add-path::/zoo\n")
|
|
a.Equal("/zoo", rc.ExtraPath[0])
|
|
|
|
handler("::add-path::/boo\n")
|
|
a.Equal("/boo", rc.ExtraPath[1])
|
|
}
|
|
|
|
func TestStopCommands(t *testing.T) {
|
|
a := assert.New(t)
|
|
ctx := context.Background()
|
|
rc := new(RunContext)
|
|
handler := rc.commandHandler(ctx)
|
|
|
|
handler("::set-env name=x::valz\n")
|
|
a.Equal("valz", rc.Env["x"])
|
|
handler("::stop-commands::my-end-token\n")
|
|
handler("::set-env name=x::abcd\n")
|
|
a.Equal("valz", rc.Env["x"])
|
|
handler("::my-end-token::\n")
|
|
handler("::set-env name=x::abcd\n")
|
|
a.Equal("abcd", rc.Env["x"])
|
|
}
|
|
|
|
func TestAddpathADO(t *testing.T) {
|
|
a := assert.New(t)
|
|
ctx := context.Background()
|
|
rc := new(RunContext)
|
|
handler := rc.commandHandler(ctx)
|
|
|
|
handler("##[add-path]/zoo\n")
|
|
a.Equal("/zoo", rc.ExtraPath[0])
|
|
|
|
handler("##[add-path]/boo\n")
|
|
a.Equal("/boo", rc.ExtraPath[1])
|
|
}
|
|
|
|
func TestAddmask(t *testing.T) {
|
|
logger, hook := test.NewNullLogger()
|
|
|
|
a := assert.New(t)
|
|
ctx := context.Background()
|
|
loggerCtx := common.WithLogger(ctx, logger)
|
|
|
|
rc := new(RunContext)
|
|
handler := rc.commandHandler(loggerCtx)
|
|
handler("::add-mask::my-secret-value\n")
|
|
|
|
a.NotEqual(" \U00002699 *my-secret-value", hook.LastEntry().Message)
|
|
}
|