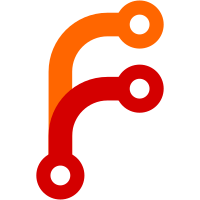
* Update compile fail tests for latest Rust The messages appear to have changed on nightly * Fix tests depending on fixture data * Fix more integration test paths * Fix doc warnings
136 lines
2.5 KiB
Rust
136 lines
2.5 KiB
Rust
#[macro_use]
|
|
extern crate bencher;
|
|
extern crate juniper;
|
|
|
|
use bencher::Bencher;
|
|
|
|
use juniper::{execute_sync, RootNode, EmptyMutation, EmptySubscription, Variables};
|
|
use juniper::tests::fixtures::starwars::model::Database;
|
|
|
|
fn query_type_name(b: &mut Bencher) {
|
|
let database = Database::new();
|
|
let schema = RootNode::new(
|
|
&database,
|
|
EmptyMutation::<Database>::new(),
|
|
EmptySubscription::<Database>::new()
|
|
);
|
|
|
|
let doc = r#"
|
|
query IntrospectionQueryTypeQuery {
|
|
__schema {
|
|
queryType {
|
|
name
|
|
}
|
|
}
|
|
}"#;
|
|
|
|
b.iter(|| execute_sync(doc, None, &schema, &Variables::new(), &database));
|
|
}
|
|
|
|
fn introspection_query(b: &mut Bencher) {
|
|
let database = Database::new();
|
|
let schema = RootNode::new(
|
|
&database,
|
|
EmptyMutation::<Database>::new(),
|
|
EmptySubscription::<Database>::new(),
|
|
);
|
|
|
|
let doc = r#"
|
|
query IntrospectionQuery {
|
|
__schema {
|
|
queryType { name }
|
|
mutationType { name }
|
|
subscriptionType { name }
|
|
types {
|
|
...FullType
|
|
}
|
|
directives {
|
|
name
|
|
description
|
|
locations
|
|
args {
|
|
...InputValue
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
fragment FullType on __Type {
|
|
kind
|
|
name
|
|
description
|
|
fields(includeDeprecated: true) {
|
|
name
|
|
description
|
|
args {
|
|
...InputValue
|
|
}
|
|
type {
|
|
...TypeRef
|
|
}
|
|
isDeprecated
|
|
deprecationReason
|
|
}
|
|
inputFields {
|
|
...InputValue
|
|
}
|
|
interfaces {
|
|
...TypeRef
|
|
}
|
|
enumValues(includeDeprecated: true) {
|
|
name
|
|
description
|
|
isDeprecated
|
|
deprecationReason
|
|
}
|
|
possibleTypes {
|
|
...TypeRef
|
|
}
|
|
}
|
|
|
|
fragment InputValue on __InputValue {
|
|
name
|
|
description
|
|
type { ...TypeRef }
|
|
defaultValue
|
|
}
|
|
|
|
fragment TypeRef on __Type {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
ofType {
|
|
kind
|
|
name
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
"#;
|
|
|
|
b.iter(|| execute_sync(doc, None, &schema, &Variables::new(), &database));
|
|
}
|
|
|
|
benchmark_group!(queries, query_type_name, introspection_query);
|
|
benchmark_main!(queries);
|