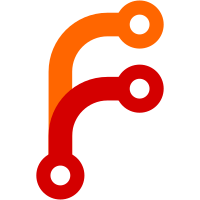
- rework `make_graphql_filter()` and `make_graphql_filter_sync()` to execute `context_extractor` only once - handle all non-recoverable `Rejection`s in `make_graphql_filter()` and `make_graphql_filter_sync()` - relax requirement for `context_extractor` to be a `BoxedFilter` only - remove `JoinError` from public API - provide example of fallible `context_extractor` in `make_graphql_filter()` API docs Additionally: - split `juniper_warp` modules into separate files - add @tyranron as `juniper_warp` co-author
37 lines
1.2 KiB
Rust
37 lines
1.2 KiB
Rust
//! [`JuniperResponse`] definition.
|
|
|
|
use juniper::{http::GraphQLBatchResponse, DefaultScalarValue, ScalarValue};
|
|
use warp::{
|
|
http::{self, StatusCode},
|
|
reply::{self, Reply},
|
|
};
|
|
|
|
/// Wrapper around a [`GraphQLBatchResponse`], implementing [`warp::Reply`], so it can be returned
|
|
/// from [`warp`] handlers.
|
|
pub(crate) struct JuniperResponse<S = DefaultScalarValue>(pub(crate) GraphQLBatchResponse<S>)
|
|
where
|
|
S: ScalarValue;
|
|
|
|
impl<S> Reply for JuniperResponse<S>
|
|
where
|
|
S: ScalarValue + Send,
|
|
{
|
|
fn into_response(self) -> reply::Response {
|
|
match serde_json::to_vec(&self.0) {
|
|
Ok(json) => http::Response::builder()
|
|
.status(if self.0.is_ok() {
|
|
StatusCode::OK
|
|
} else {
|
|
StatusCode::BAD_REQUEST
|
|
})
|
|
.header("content-type", "application/json")
|
|
.body(json.into()),
|
|
Err(e) => http::Response::builder()
|
|
.status(StatusCode::INTERNAL_SERVER_ERROR)
|
|
.body(e.to_string().into()),
|
|
}
|
|
.unwrap_or_else(|e| {
|
|
unreachable!("cannot build `reply::Response` out of `JuniperResponse`: {e}")
|
|
})
|
|
}
|
|
}
|