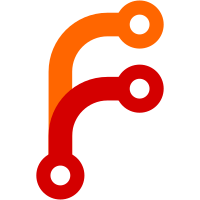
- reimplement #[derive(GraphQLUnion)] macro to support: - both structs and enums - generics in type definition - multiple #[graphql] attributes - external resolver functions - remove From trait impls generation for enum variants - reimplement #[graphql_union] macro to support: - traits - generics in trait definition - multiple attributes - external resolver functions - GraphQLType implemetation for a raw trait object - GraphQLTypeAsync implemetation (#549) - add marker::GraphQLUnion trait - rewrite "2.5 Unions" section in Book (Juniper user documentation) - rewrite `codegen` and `codegen_fail` integration tests for GraphQL unions Additionally: - re-export `futures` crate in `juniper` for convenient reuse in the generated code without requiring library user to provide `futures` crate by himself (#663) - use unit type () as default context for EmptyMutation and EmptySubscriptions - relax Sized trait bound on some GraphQLType and GraphQLTypeAsync definitions, implementations and usages
96 lines
1.9 KiB
Rust
96 lines
1.9 KiB
Rust
use std::{
|
|
hash::{Hash, Hasher},
|
|
ops,
|
|
};
|
|
|
|
use proc_macro2::{Span, TokenStream};
|
|
use quote::ToTokens;
|
|
|
|
#[derive(Clone, Debug)]
|
|
pub struct SpanContainer<T> {
|
|
expr: Option<Span>,
|
|
ident: Span,
|
|
val: T,
|
|
}
|
|
|
|
impl<T: ToTokens> ToTokens for SpanContainer<T> {
|
|
fn to_tokens(&self, tokens: &mut TokenStream) {
|
|
self.val.to_tokens(tokens)
|
|
}
|
|
}
|
|
|
|
impl<T> SpanContainer<T> {
|
|
pub fn new(ident: Span, expr: Option<Span>, val: T) -> Self {
|
|
Self { ident, expr, val }
|
|
}
|
|
|
|
pub fn span_ident(&self) -> Span {
|
|
self.ident
|
|
}
|
|
|
|
pub fn span_joined(&self) -> Span {
|
|
if let Some(s) = self.expr {
|
|
// TODO: Use `Span::join` once stabilized and available on stable:
|
|
// https://github.com/rust-lang/rust/issues/54725
|
|
// self.ident.join(s).unwrap()
|
|
|
|
// At the moment, just return the second, more meaningful part.
|
|
s
|
|
} else {
|
|
self.ident
|
|
}
|
|
}
|
|
|
|
pub fn into_inner(self) -> T {
|
|
self.val
|
|
}
|
|
|
|
pub fn inner(&self) -> &T {
|
|
&self.val
|
|
}
|
|
|
|
pub fn map<U, F: Fn(T) -> U>(self, f: F) -> SpanContainer<U> {
|
|
SpanContainer {
|
|
expr: self.expr,
|
|
ident: self.ident,
|
|
val: f(self.val),
|
|
}
|
|
}
|
|
}
|
|
|
|
impl<T> AsRef<T> for SpanContainer<T> {
|
|
fn as_ref(&self) -> &T {
|
|
&self.val
|
|
}
|
|
}
|
|
|
|
impl<T> ops::Deref for SpanContainer<T> {
|
|
type Target = T;
|
|
|
|
fn deref(&self) -> &Self::Target {
|
|
&self.val
|
|
}
|
|
}
|
|
|
|
impl<T: PartialEq> PartialEq for SpanContainer<T> {
|
|
fn eq(&self, other: &Self) -> bool {
|
|
self.val == other.val
|
|
}
|
|
}
|
|
|
|
impl<T: Eq> Eq for SpanContainer<T> {}
|
|
|
|
impl<T: PartialEq> PartialEq<T> for SpanContainer<T> {
|
|
fn eq(&self, other: &T) -> bool {
|
|
&self.val == other
|
|
}
|
|
}
|
|
|
|
impl<T: Hash> Hash for SpanContainer<T> {
|
|
fn hash<H>(&self, state: &mut H)
|
|
where
|
|
H: Hasher,
|
|
{
|
|
self.val.hash(state)
|
|
}
|
|
}
|